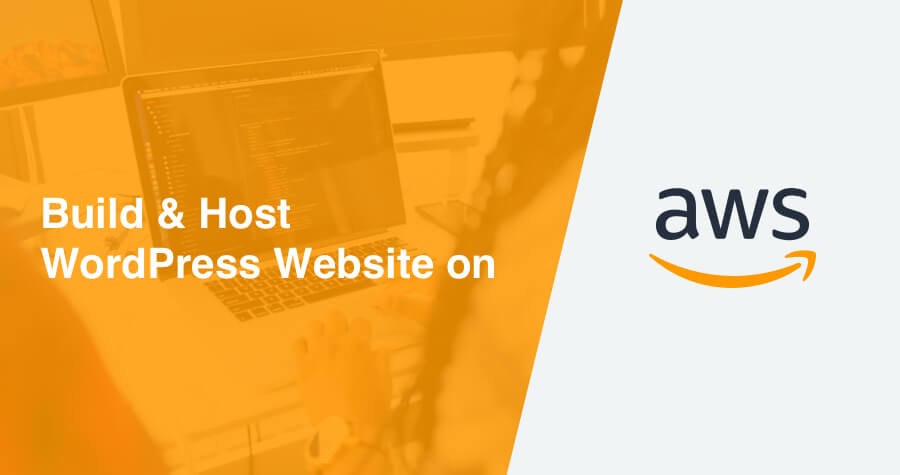
Sep 9 2015
Are you searching for the best PHP frameworks to use?PHP is used by 82% of majority web server and its frameworks are widely popular among developers....
View Blog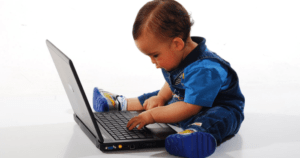
Sep 15 2014
Do you want to learn some of the best practices of PHP? Check out this post as it attempts to teach you the best practices of the most widely used pro...
View Blog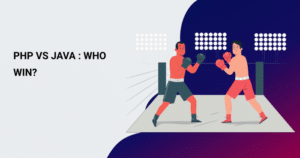
Sep 23 2013
Many people ask me almost every week or so: Which one is better between PHP and Java? I come with their solution and discussed each of important point...
View Blog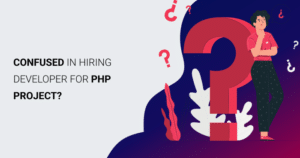
Sep 18 2013
Do you have a PHP project? Are you searching for talented PHP professional? Read this article and follows 6 essential points while choosing dedicated ...
View Blog